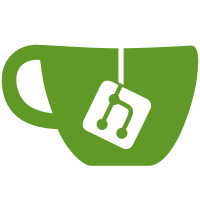
it turned out that a hashmap isn't the right datastructure, as the special-case header Set-Cookie not only can, but is even heavily recommended to be used multiple times. we now use a dumb list as a key-value store for this purpose, but restrict it to max 256 entries so the linear search can always be completed in reasonable time in case of an attack. closes #403
23 lines
495 B
C
23 lines
495 B
C
#ifndef PSEUDOMAP_H
|
|
#define PSEUDOMAP_H
|
|
|
|
#include <stdlib.h>
|
|
#include "sblist.h"
|
|
|
|
struct pseudomap_entry {
|
|
char *key;
|
|
char *value;
|
|
};
|
|
|
|
typedef sblist pseudomap;
|
|
|
|
pseudomap *pseudomap_create(void);
|
|
void pseudomap_destroy(pseudomap *o);
|
|
int pseudomap_append(pseudomap *o, const char *key, char *value );
|
|
char* pseudomap_find(pseudomap *o, const char *key);
|
|
int pseudomap_remove(pseudomap *o, const char *key);
|
|
size_t pseudomap_next(pseudomap *o, size_t iter, char** key, char** value);
|
|
|
|
#endif
|
|
|